Neo4j Vector Index and Search
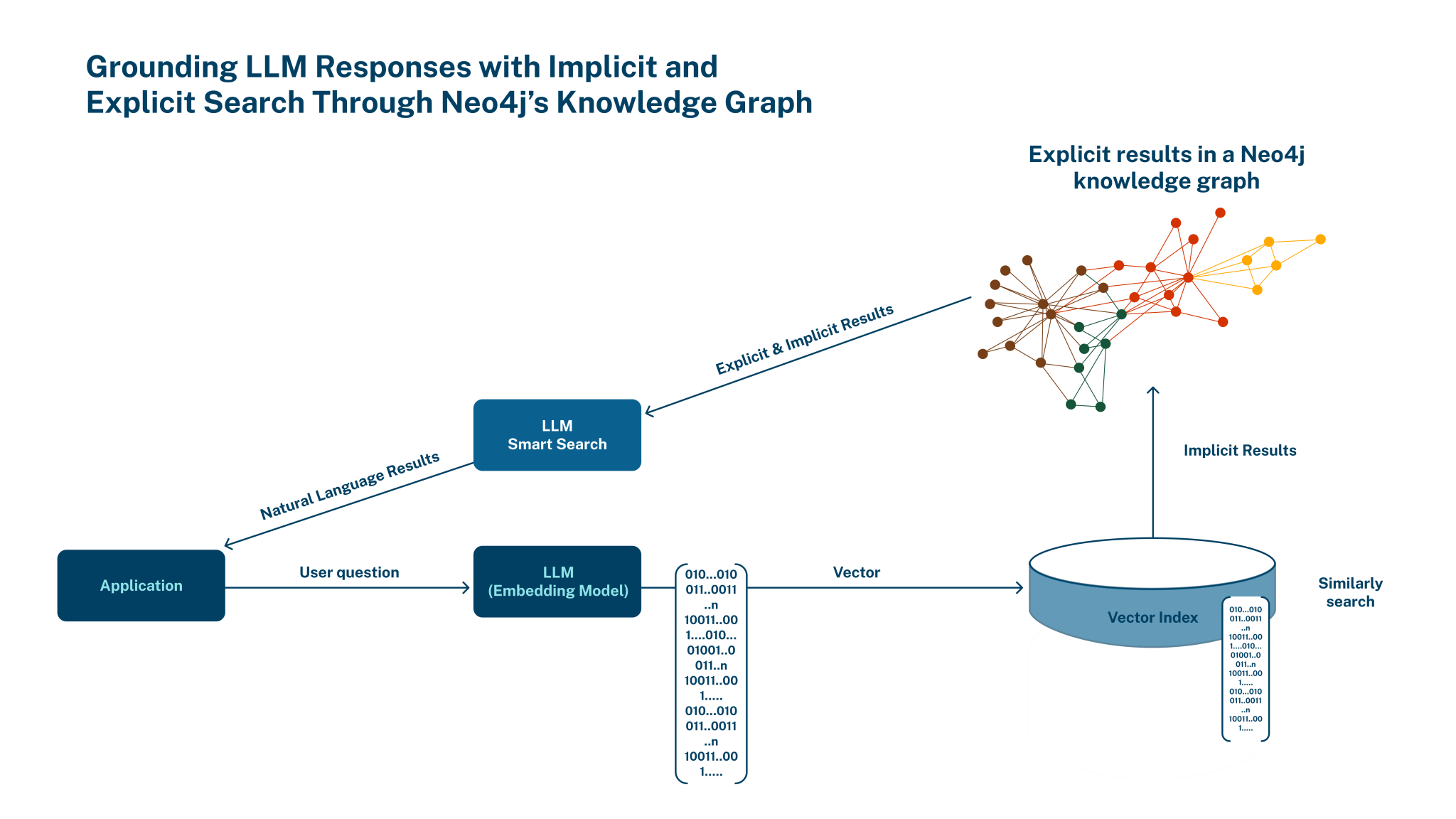
The Neo4j Vector index implements HNSW (Hierarchical Navigatable Small World) for creating layers of k-nearest neighbors to enable efficient and robust approximate nearest neighbor search. The index is designed to work with embeddings, such as those generated by machine learning models, and can be used to find similar nodes in a graph based on their embeddings.
Functionality Includes
-
Create a vector index with a specified number of dimensions and similarity function (euclidean, cosine)
-
Query vector index with embedding and top-k, returning nodes and similarity score
-
Procedures to compute text vector embeddings with OpenAI, AWS Bedrock, Google Vertex AI, and other ML platforms
-
Vector similarity functions to compute cosine angle and euclidean distance between vectors
Usage
// create vector index
CREATE VECTOR INDEX `abstract-embeddings`
FOR (n: Abstract) ON (n.embedding)
OPTIONS {indexConfig: {
`vector.dimensions`: 1536,
`vector.similarity_function`: 'cosine'
}};
// set embedding as parameter
MATCH (a:Abstract {id: $id})
CALL db.create.setNodeVectorProperty(a, 'embedding', $embedding);
// use a genai function to compute the embedding
MATCH (a:Abstract {id: $id})
WITH a, genai.vector.encode(a.text, "OpenAI", { token: $token }) AS embedding
CALL db.create.setNodeVectorProperty(n, 'embedding', embedding);
// query vector index for similar abstracts
MATCH (title:Title)<--(:Paper)-->(abstract:Abstract)
WHERE title.text CONTAINS = 'hierarchical navigable small world graph'
CALL db.index.vector.queryNodes('abstract-embeddings', 10, abstract.embedding)
YIELD node AS similarAbstract, score
MATCH (similarAbstract)<--(:Paper)-->(similarTitle:Title)
RETURN similarTitle.text AS title, score;
// use cosine similarity for exact nearest neighbor search
// pre-filter vector search
MATCH (venue:Venue)<--(:Paper)-->(abstract:Abstract)
WHERE venue.name CONTAINS 'NETWORK'
WITH abstract, paper,
vector.similarity.cosine(abstract.embedding, $embedding) AS score
WHERE score > 0.9
RETURN paper.title AS title, abstract.text, score
ORDER BY score DESC LIMIT 10;